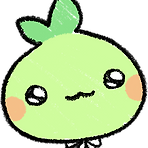
[#1. JAVA의 특징] 1. 간결한 프로그래밍 문법 - C/C++에서 머리 빠개지는 포인터 개념이 없다 - Garbage Collector가 메모리 관리 알아서 처리 2. 이식성이 높다 - JVM(Java Virtual Machine) 사용. 운영체제에 영향받지 않는다. = 플랫폼에 독립적. - Write Once, Run Anywhere 3. 객체지향 언어 - OOP(Object Oriented Programming) - 캡슐화, 상속, 다형성 4. 멀티 쓰레드 구현, 병렬 처리 5. 다양한 응옹 프로그램 작성 가능 - 애플릿, JSP, 서블릿, 임베디드 프로그램, 모바일 앱 등등.. 6. 오픈 소스 라이브러리 풍부 ★ # JRE와 JDK JRE(Java Runtime Enviroment) JDK(..
[객체 지향 프로그래밍, Object-Oriented Programming, OOP] "객체 지향 프로그래밍은 컴퓨터 프로그램들을 명령어의 목록에서 보는 시각에서 벗어나 여러개의 독립된 단위, 즉 "객체"들의 모임으로 파악하고자 하는 것이다. 각각의 객체는 메시지를 주고받고, 데이터를 처리할 수 있다" "객체 지향 프로그래밍은 프로그램을 대규모 소프트웨어 개발에 많이 사용된다." -> 컴퓨터 부품을 갈아끼듯이 객체를 필요에 따라 유연하게 변경하면서 개발할 수 있는 프로그래밍 방법. 이라고 하는데, 이정도로는 객체지향을 이해하기에 30%정도 부족한 느낌이다. 자바 스프링의 대가 김영한 강사님이 추천해주신 책을 읽고 나서 객체지향이란 대체 멀까에 대해 정리해 보고자 한다. 1. 객체지향이란? 객체지향은 현실..
# 버블정렬 | O(N^2) public static void bubbleSort(int[] arr) { // O(n^2) for(int i = 0; i arr[j + 1]) { swap(arr, j, j + 1); } } } } # 선택정렬 | O(N^2) public static void selectedSort(int[] arr) { // O(n^2) for(int i = 0; i < arr.length - 1; i++) { int minidx = i; for(int j = i + 1; j < arr.length; j++) { if(arr[j] < arr[min..
# 제네릭 스택(연결리스트 활용) package StudyJava.Stack; import java.util.EmptyStackException; public class GenericStack { class Node { private T data; private Node next; public Node(T data) { this.data = data; } } private Node top; public T pop() { if(top == null) { throw new EmptyStackException(); } T item = top.data; top = top.next; return item; } public void push(T item) { Node t = new Node(item); t.next..
# 각 자료형의 초깃값 byte int long double char boolean 참조형 (byte)0 0 0L 0.0d '\u0000' false 공백 or NULL - 배열을 선언하면 배열의 구성요소는 자동으로 0으로 초기화 된다. - 메소드 내부에서 선언된 지역변수는 초깃값으로 초기화되지 않는다 # 접근 제한자 public: 모든 접근 허용 protected: 같은 패키지의 객체, 상속 관계의 객체 허용 default: 같은 패키지의 객체 허용 private: 현재의 객체 안에서만 사용 클래스: public, default 생성자: public, protected, default, private 멤버 변수: public, protected, default, private 멤버 메소드: public..
도서관에 반밖에 못본 SQL 책 반납하러 갔는데 반납한 책이 당일대출이 안되는 바람에 SQL 책을 못빌려왔다.. 어쩔 수 없이 다른 책을 찾아보는 중에 자바 알고리즘 입문책이 있어서 이거라도 정리해보기로 했다. 입문 난이도라서 1주면 뗄수 있지 않을까 기대하면서 시작! 자료형 메서드 범위 boolean nextBoolean() true/false byte nextBite() -128 ~ 127 short nextShort() -32768 ~ 32767 int nextInt() -2,147,483,648 ~ 2,147,483,647 long nextLong() 개큼 float nextFloat() 조금 큼 double nextDouble() 개큼 String next() 문자열(스페이스, 개행문자로 구분)..
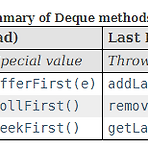
https://docs.oracle.com/en/java/javase/11/docs/api/java.base/java/util/Deque.html Deque (Java SE 11 & JDK 11 ) A linear collection that supports element insertion and removal at both ends. The name deque is short for "double ended queue" and is usually pronounced "deck". Most Deque implementations place no fixed limits on the number of elements they may contain, bu docs.oracle.com A linear colle..
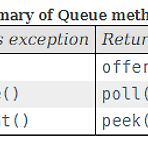
https://docs.oracle.com/en/java/javase/11/docs/api/java.base/java/util/Queue.html#method.summary Queue (Java SE 11 & JDK 11 ) A collection designed for holding elements prior to processing. Besides basic Collection operations, queues provide additional insertion, extraction, and inspection operations. Each of these methods exists in two forms: one throws an exception if the opera docs.oracle.com..